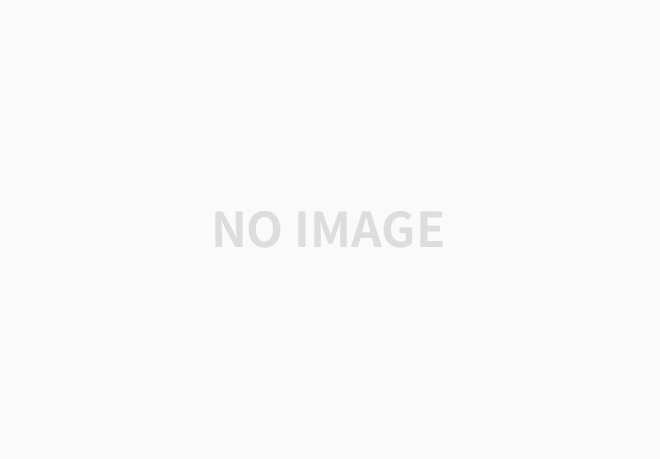
Introduction
The token bucket algorithm is a useful tool for allocating resources by helping to regulate the rate of requests sent to a server. In this blog post, I'll explain how the token bucket algorithm works and provide a sample code written in JavaScript.
What is the Token Bucket Algorithm?
The token bucket algorithm is a method of regulating a data stream sent to a server. It works by assigning a token to a bucket, which is essentially a buffer that stores a set number of tokens. When the bucket is full, it can send a request to the server. When the request is sent, the number of tokens in the bucket is reduced. The bucket will then fill up again over time, allowing for more requests to be sent.
Sample Code in JavaScript
Below is a sample code of the token bucket algorithm written in JavaScript.
// Create a bucket with a capacity of 10 tokens
let tokenBucket = {
capacity: 10,
tokens: 10
}
// Function to send a request to the server
function sendRequest() {
if (tokenBucket.tokens > 0) {
// If the bucket has tokens, send the request
tokenBucket.tokens -= 1;
console.log("Request sent!");
} else {
// If the bucket is empty, wait until it has tokens
console.log("Bucket is empty. Waiting to send request.");
}
}
// Function to add tokens to the bucket
function addTokens() {
if (tokenBucket.tokens < tokenBucket.capacity) {
// If the bucket is not full, add tokens to it
tokenBucket.tokens += 1;
console.log("Token added to bucket!");
} else {
// If the bucket is full, do nothing
console.log("Bucket is full. No tokens added.");
}
}
Conclusion
The token bucket algorithm is a useful way to regulate the rate of requests sent to a server. With the help of the sample code provided, you can now implement the algorithm in your own JavaScript projects.
Written by Notion AI
'컴퓨터 공학 > JavaScript' 카테고리의 다른 글
TCO (Tail Call Optimization) 개념 및 예제 (0) | 2023.01.14 |
---|---|
pkg 모듈 실행 시 "(0, assert_1.default)(!this.bar)" 에러 발생하는 경우 (0) | 2022.12.04 |
VSCode에서 JavaScript CommonJS의 export 객체 속성들 <Find Reference>에 검색되지 않는 문제 (1) | 2022.09.06 |
Cloudflare Workers와 Notion API로 유저 데이터 관리하기 (0) | 2022.08.14 |
JavaScript로 interface 모사하기 (0) | 2022.08.06 |